Welcome back to another C tutorial, this post covering the first part of control structures, if statements. Let's get right into it.
What Is an if Statement?
If statements are conditionals which allow the programmer to direct execution flow depending on certain circumstances, for example, the value of a variable. It's a pretty straight-forward concept, so let's write some code.
Example Code
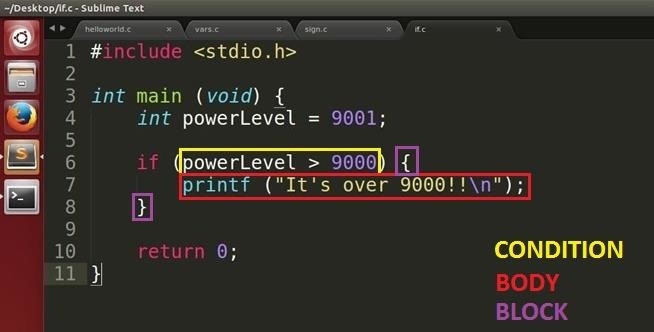
As you can see, creating an if statement is a cinch. All you need is the "if" followed by a condition and then a pair of curly braces to create a block where execution will continue. In this case, the condition I have given is if the value in variable "powerLevel" is greater than the value 9000, it will print "It's over 9000!!", otherwise, it will skip the if block and continue execution after it.
Compiling and Running
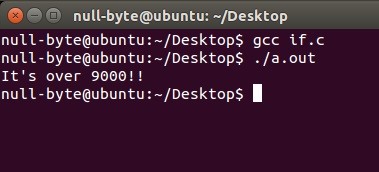
Results are as expected.
If-Else
In addition to the simple if statement, there is a method to give another path of execution, i.e. the else statement. If you have not already figured out what this self-explanatory statement does, it gives the control flow something to fall back upon provided the initial if condition fail.
Example Code
This code shows you how the else statement is written. It is unlike the if statement since it does not have a condition to satisfy as it acts as a sort of net where the program flow will fall back upon as I explained above.

Now our powerLevel is set to 9000, let's compile and run this to see the result.
Compiling and Running
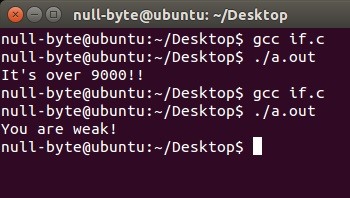
Since 9000 is not greater than 9000, it will skip the initial if block and fall back upon the else block as it should. Nothing out of the ordinary here.
If-Else If
Again, another neat addition onto this is the else if statement which provides the programmer the ability to create multiple if conditions should any fail, allowing a more flexible control of the execution path. Let's look at another example.
Example Code

As you can see, the else if statement requires its own condition, similar to the if statement but unlike the else statement. Once one of either the if statement's or an else if statement's block of code has finished execution, it will jump out and continue after the end of the whole if, else if and else section. What will you expect to happen when we compile and run? Let's find out.
Compiling and Running

If you expected anything different, then there's an issue. If you ask, "Why didn't it print out "You are worthy!"?" The answer to that is because execution goes from the top and flows downwards. Since the condition that powerLevel is greater than 42 was approached and satisfied first, it will jump into that block and when it's done, jump out and over the rest of the else if sections. Please note this and be careful with prioritizing your code.
Conclusion
This concludes the if statements and we shall move onto loops in the next tutorial. Play around and experiment a bit, try other data types such as characters and see if you can find out how to write conditionals for them. Until next time.
dtm.
Just updated your iPhone? You'll find new emoji, enhanced security, podcast transcripts, Apple Cash virtual numbers, and other useful features. There are even new additions hidden within Safari. Find out what's new and changed on your iPhone with the iOS 17.4 update.
Be the First to Comment
Share Your Thoughts